MVC vs MVP vs MVVM vs MVI. Choosing an Architecture for Android App
Before you build a house, you need to lay a foundation. The type of foundation will depend on how big your house will be, how many stories it will have, and what materials you’ll use to build it. The same goes for mobile software: before you start developing it, you need to create an architecture. The architecture will influence your app’s scalability and overall structure.
Challenges of building an architecture
There’s no perfect architecture. Each project is unique, with its own requirements. The things that influence the architecture are the same for Android and iOS. In this article we’ll focus on architecture for Android app development.
These are the things that influence the architecture:
- Technology stack
- Tool set, server infrastructure, databases and SDKs
- Usage of cloud technologies
- Integration of third-party services like maps, payment gateways, etc.
- Future plans
- Type of project
- Expertise of the developers
As you can see, it’s important to consider and sometimes even predict circumstances that may arise in the future.
The mobile app market is dynamic, and nobody can tell for sure what will happen next. New programming languages, services, libraries, analytics and operating system versions appear every year. A mobile expert will create an app architecture with this in mind.
The process of choosing the right android mobile application architecture always causes passionate discussion among developers who often have different views
The good news is that the mobile world is becoming more standardized, and big companies like Google are making sure the transition from old tools and operating systems to new ones is easy for developers.
The process of choosing the right android mobile application architecture always causes passionate discussion among developers who often have different views.
This is because the choice partly depends on previous experience of the developer and their personal preferences. This topic is complex, but in this article we’ll take a pragmatic approach and discuss what each architecture has to offer.
What Determines a Good Android Mobile Application Architecture?
What do we want to achieve when we design an architecture? There are four things every developer wants to achieve with their architecture.
- Scalability
- Reliability
- Maintainability
- Code reusability and testability
Scalability
Scalability allows you to expand your project as your business grows. It means that the project can handle new features and rapid growth of the user base. Scalability can be compared with adding new stories to your house.
If the foundation was built for a one-storey house, you won’t be able to add a second floor. The same works with an app’s architecture: if you don’t build your app with growth in mind, you’ll have a hard time adding features that can benefit your business and help you keep up with competitors.
Refactoring the app to fit the extra features will take way more time and money than it would have if the architecture was well-thought-out initially
Developers will certainly be able to add features to an app that wasn’t designed to handle them. But they’ll need to use duct tape to make it work. Sometimes backend doesn’t fit as well. Naturally, this will lead to inconsistencies in code, resulting in bugs and crashes. Each time a developer tries to fit in another feature, the technical debt will grow.
In the end, the whole app will require fundamental refactoring. This means developers will need to rebuild the architecture for Android mobile application from scratch and refactor all features they implemented previously. In the end, this will take way more time and money than it would have if the architecture was well-thought-out initially.
Reliability
Reliability also depends on the architecture, as it defines how parts of the app interact with each other. Stable applications without major inconsistencies in code can only exist with a suitable architecture.
Reliability also depends on the scalability of an app. If an app’s architecture can’t handle all the bulkheads, it will be unstable and unreliable. This will lead to bugs, crashes, and customer dissatisfaction at best and to serious security breaches at worst.
Maintainability
Work on your app doesn’t end with its release. New libraries and operating systems are constantly coming out, and keeping an app up to date is a necessity.
As your business grows and changes, you need to add new features and change the interface according to your business design and current trends on the mobile market.
Separation of concerns makes app parts independent from each other and helps to support scalability and maintainability.
Maintenance is something you don’t want to spend loads of time and money on. The easier it is to maintain your app, the less money you’ll spend.
Code reusability and testability
Code reusability is important if you want to avoid rework and save time on your project. The core principle that makes code reusable is separation of concerns.
Separation of concerns means that different ideas should be separated in the code. For example, you should describe the logic of uploading images in only one place. If you want to change this functionality, you then won’t need to worry about it affecting all other parts of your app. Additionally, this makes it possible to just take a piece of code and use it elsewhere.
Testability also becomes easier if your app’s architecture is built according to the separation of concerns. A QA engineer doesn’t need to test the whole application each time to check if new functionality works.
Separation of concerns makes app parts independent from each other and helps to support scalability and maintainability.
Now that we’ve described the core principles and goals of any architecture, let’s talk about each type of architecture in detail.
Model–View–Controller
Model–View–Controller is a popular type of architecture that divides all parts of an application by purpose according to the separation of concerns principle. According to this architecture, developers organize functionality into three major categories:
- Model – Stores all the information your app contains. The model defines terms your application operates.
- View – Responsible for how your app looks. The view contains the UI of your app.
- Controller – Tells the view how to behave. The controller holds the business logic of your application.
There are two options within the MVC architecture: a supervising controller and a passive view. With a supervising controller, the view interacts with the model without the participation of the presenter. With a passive view, the presenter updates the view that reflect changes in the model.
In mobile development, a supervising controller is almost never used. Here’s the diagram that demonstrates how MVC works.
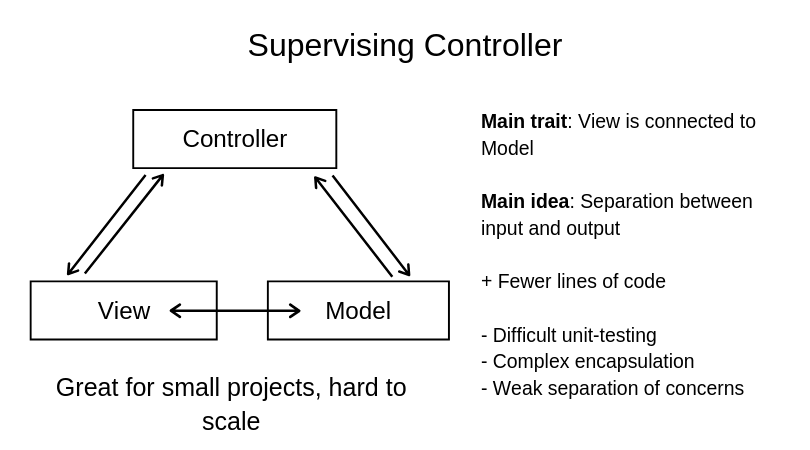
Here, you can see the pattern of the MVC with a supervising controller. The view reflects both users and reverse requests. The controller receives incoming data and the view reflects the output. Both of these parts exchange information between each other.
If choosing between MVC vs MVVM, consider the project size. MVC is a perfect architecture for small projects, but it has a rather simple structure that won’t cover all the peculiarities of a big project, for example an enterprise app or an app for healthcare or banking.
Model–View–Presenter
The Model–View–Presenter (MVP) architecture makes it easy to test an application. The presenter part of the application can be reused many times, as it’s able to represent several interfaces.
Model–View–Presenter also tries to comply with the separation of concerns principle. It separates the interface of the app (the view) from the model, and it’s mostly used to build user interfaces. The presenter in this architecture works as an intermediary between the view and the model.
However, to successfully build an interface, you should use MVP or MVC as your inspiration, not apply these development patterns directly.
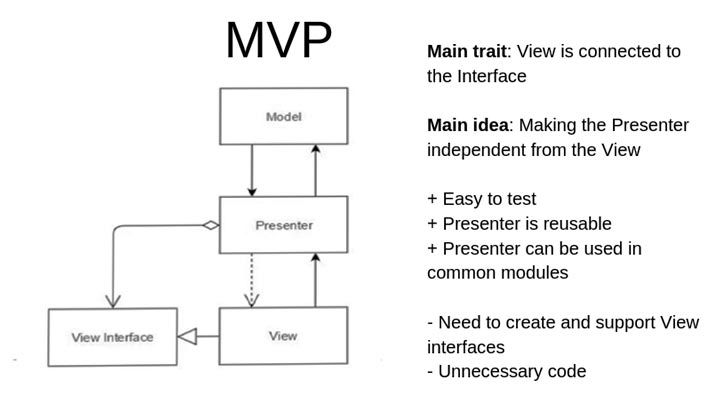
There are two elements developers use to make the MVP architecture work effectively: use cases and view bindings. Let’s talk about them in detail.
Use cases
Creating use cases means organizing the business logic into separate classes and making them part of the model. These classes are independent from the controller, and each class contains one rule of business logic. This makes it easier to use these classes several times and write tests for them.
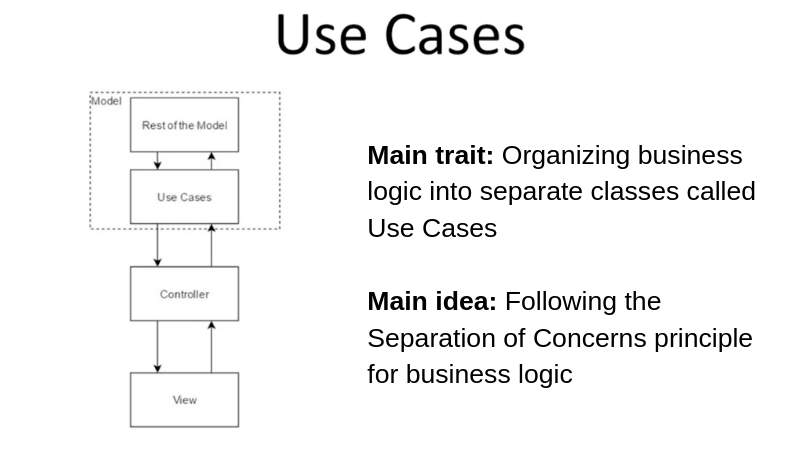
In this example on GitLab, you can see how a validation use case and a login use case are organized into a login controller. A login controller connects the app to the network. If there are common business logic rules in other controllers and presenters, you’ll be able to use these ready use cases for other parts of your mobile solution.
Data bindings
There are four linear functions in the MVP architecture that don’t do anything except make minor changes to the user interface. To avoid using extra code, you can use view binding.
View binding, or data binding, is the process of creating little reusable pieces of a user interface. All you need to do is write code in XML and all the communication with the components is done by the bindings.
This makes your code clean and relieves you from stress and unnecessary code. Data binding is great for huge projects, as it saves time and makes your project easier to read and maintain.
You can find examples of binding here.
Model–View–ViewModel
Model–View–ViewModel (MVVM) is another architecture. At first glance it reminds you of the MVP pattern, as they both separate the view from the business logic. There’s a core difference though. While MVP makes the view independent from a specific user interface platform, MVVM is focused on event-driven programming of user interfaces. This means that MVVM works great when you need to make fast design changes.
Model–View–ViewModel architecture consists of:
- Model – Represents either a real state content, or a data access layer that represents content.
- View – Reflects user actions and informs the ViewModel
- ViewModel – Transfers data according to what the View requests
In the MVP pattern, the presenter tells the view what to show to the user. In MVVM, the ViewModel streams events that the View can bind to. So there’s no need for the ViewModel to address the View.
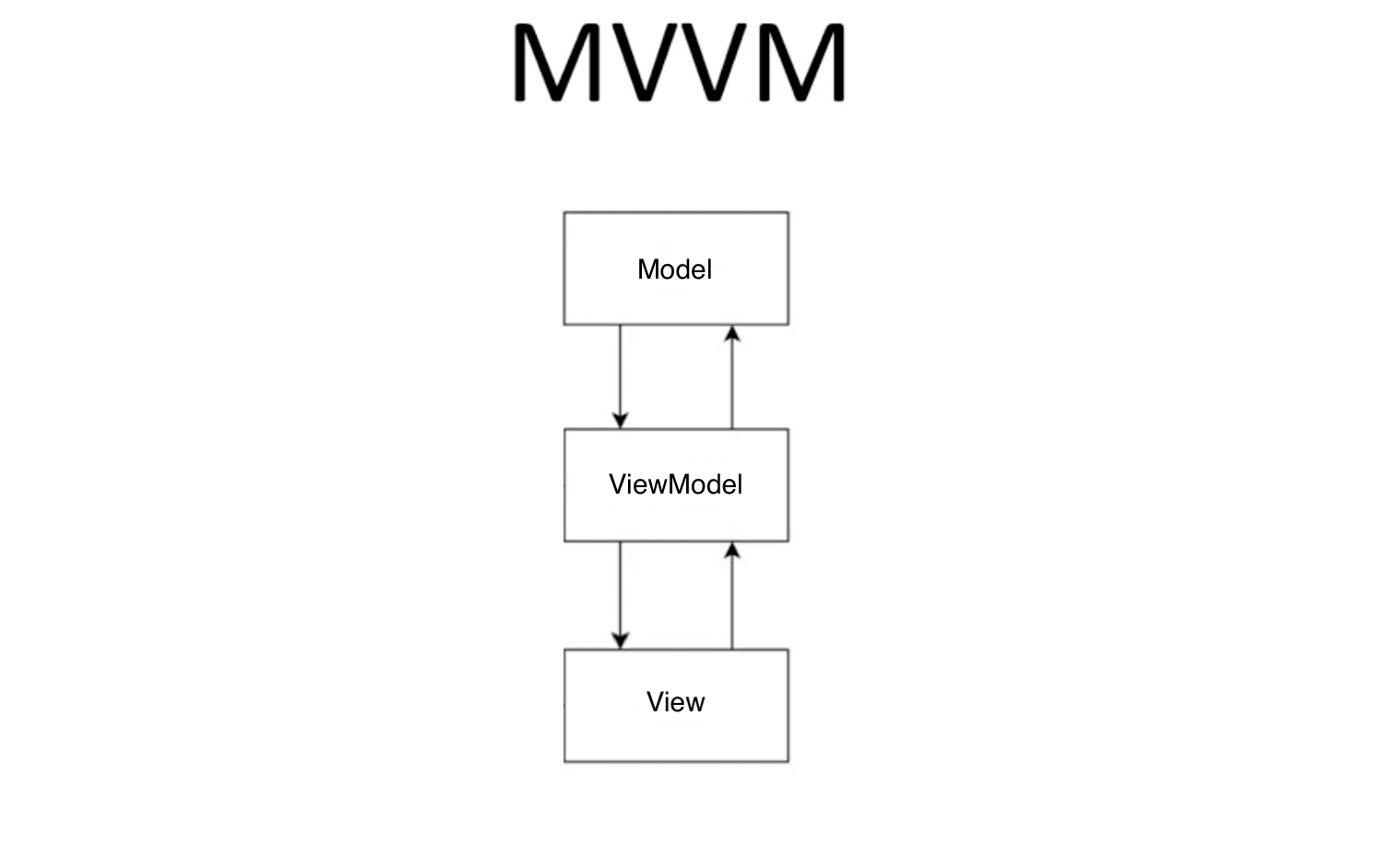
Model–View–Intent
Another architecture you can use for your mobile application is Model–View–Intent, or MVI. MVI can only work with RxJava – a Reactive programming library.
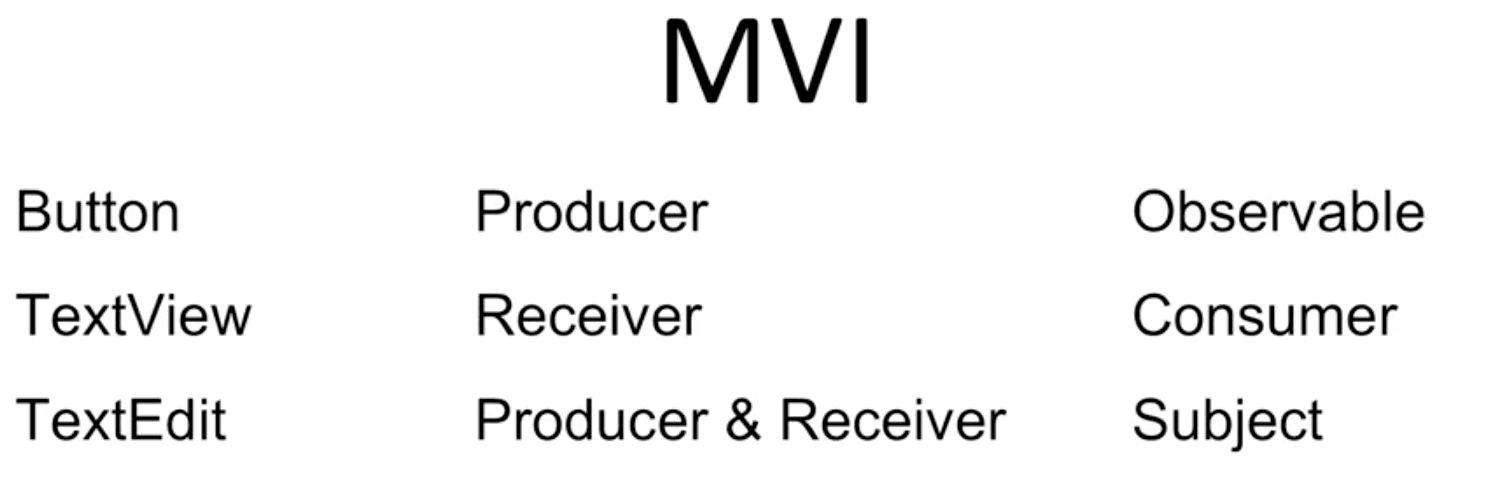
MVI is based on data streams that connect different elements which are present in the project according to RxJava terminology. The main idea of the MVI development pattern is that no element of the layout does anything but send or receive data, and elements are divided according to what role they have in this data exchange.
There are three main groups of elements in RxJava:
- Observable
- Consumer
- Subject
For example, a button just tells the system if it’s pressed. This action is called an intent: it triggers an event, for example data transfer.
In the RxJava library, this event creation is Observable. In the paradigm of reactive development, a button is Observable.
On the other hand, there are some elements that don’t create any data or perform any actions: for example, TextView. In RxJava, such elements that only receive data are called Consumer elements.
Another type of element (for example, TextEdit) can both receive and send data. This element is both a producer and a receiver of data at the same time. It is called a Subject in RxJava.
In MVI, everything is a data stream that starts when a producer releases data and ends when a receiver gets that data.
Final thoughts
Although the separation of concerns principle requires some effort at the initial stage of mobile app development, it’s a great way to make your code high-quality, scalable, understandable, and reliable.
Development patterns like MVVM and MVI can help to improve the scalability of your mobile app, but note that they’re dependent on RxJava.
Use a combination of architecture patterns so that it fits the unique needs and goals of your mobile application
When deciding on an android architecture in mobile application development, remember that you don’t have to choose only one. Each project is unique, and to make a custom architecture that will reflect and support its needs, you can take several architectures and use elements from them, for example combining MVC and MVVM.
All you need to create your own custom mobile app architecture is an experienced tech lead who’s able to see current and future needs of your project and business and create an architecture according to your goals and technology stack.